https://youtu.be/zpzhHikKElo?feature=shared
BoardController에 게시글 상세 조회 기능 구현
package test.board.Controller;
import lombok.RequiredArgsConstructor;
import org.springframework.data.domain.Pageable;
import org.springframework.data.web.PageableDefault;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.*;
import test.board.DTO.BoardDTO;
import test.board.Service.BoardService;
import java.io.IOException;
import java.util.List;
@Controller
@RequiredArgsConstructor
@RequestMapping("/board")
public class BoardController {
private final BoardService boardService;
@GetMapping("/save")
public String saveForm() {
return "save";
}
@PostMapping("/save")
public String save(@ModelAttribute BoardDTO boardDTO) throws IOException {
System.out.println("boardDTO = " + boardDTO);
boardService.save(boardDTO);
return "index";
}
@GetMapping("/")
public String findAll(Model model) {
List<BoardDTO> boardDTOList = boardService.findAll();
model.addAttribute("boardList", boardDTOList);
return "list";
}
@GetMapping("/{id}")
public String findById(@PathVariable Long id, Model model,
@PageableDefault(page=1) Pageable pageable) {
/*
해당 게시글의 조회수를 하나 올리고
게시글 데이터를 가져와서 detail.html에 출력
*/
boardService.updateHits(id);
BoardDTO boardDTO = boardService.findById(id);
model.addAttribute("board", boardDTO);
return "detail";
}
}
BoardService
@Transactional
public void updateHits(Long id) {
boardRepository.updateHits(id);
}
BoardRepository
package test.board.Repository;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.jpa.repository.Modifying;
import org.springframework.data.jpa.repository.Query;
import org.springframework.data.repository.query.Param;
import test.board.Entity.BoardEntity;
public interface BoardRepository extends JpaRepository<BoardEntity, Long> {
// update board_table set board_hits=board_hits+1 where id=?
@Modifying
@Query(value = "update BoardEntity b set b.boardHits=b.boardHits+1 where b.id=:id")
void updateHits(@Param("id") Long id);
}
BoardService
@Transactional
public BoardDTO findById(Long id) {
Optional<BoardEntity> optionalBoardEntity = boardRepository.findById(id);
if (optionalBoardEntity.isPresent()) {
BoardEntity boardEntity = optionalBoardEntity.get();
BoardDTO boardDTO = BoardDTO.toBoardDTO(boardEntity);
return boardDTO;
} else {
return null;
}
}
detail.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>detail</title>
<!-- jquery cdn -->
<script src="https://code.jquery.com/jquery-3.6.3.min.js" integrity="sha256-pvPw+upLPUjgMXY0G+8O0xUf+/Im1MZjXxxgOcBQBXU=" crossorigin="anonymous"></script>
</head>
<body>
<table>
<tr>
<th>id</th>
<td th:text="${board.id}"></td>
</tr>
<tr>
<th>title</th>
<td th:text="${board.boardTitle}"></td>
</tr>
<tr>
<th>writer</th>
<td th:text="${board.boardWriter}"></td>
</tr>
<tr>
<th>date</th>
<td th:text="${board.boardCreatedTime}"></td>
</tr>
<tr>
<th>hits</th>
<td th:text="${board.boardHits}"></td>
</tr>
</table>
<button onclick="listReq()">목록</button>
<button onclick="updateReq()">수정</button>
<button onclick="deleteReq()">삭제</button>
</body>
<script>
const listReq = () => {
console.log("목록 요청");
const page = [[${page}]];
location.href = "/board/paging?page="+page;
}
const updateReq = () => {
console.log("수정 요청");
const id = [[${board.id}]];
location.href = "/board/update/" + id;
}
const deleteReq = () => {
console.log("삭제 요청");
const id = [[${board.id}]];
location.href = "/board/delete/" + id;
}
</script>
</html>

글을 하나 더 추가할게요

이렇게 전에 작성한 글의 조회수가 올라가고 새로 작성한 조회수는 0인 것을 확인할 수 있습니다.
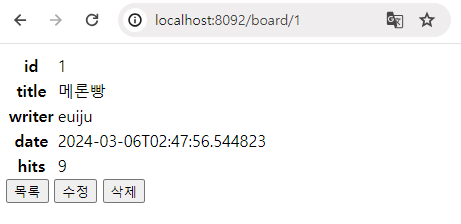
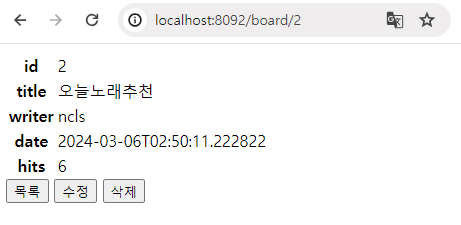
title을 눌러 게시글을 상세 조회할 수 있습니다. 게시글을 클릭할수록 조회수도 올라갑니다.

데이터베이스에도 그대로 반영됩니다.
'SpringBoot' 카테고리의 다른 글
[3] IntelliJ 스프링부트 게시판_목록 출력하기 (0) | 2024.02.13 |
---|---|
[2] IntelliJ 스프링부트 게시판_게시글 작성 (0) | 2024.02.12 |
[1] IntelliJ 스프링부트 application.yml 설정하기 (0) | 2024.02.08 |